我们先从一个例子来了解生命周期函数
一、例子2秒内透明
需求:内容在2秒内渐变透明,透明后马上回到1
<!Doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello World</title>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="test"></div>
<!--引入React 核心库-->
<script type="text/javascript" src="https://cdn.bootcss.com/react/16.4.0/umd/react.development.js"></script>
<!--引入React-dom 用于支持React操作DOM-->
<script type="text/javascript" src="https://cdn.bootcss.com/react-dom/16.4.0/umd/react-dom.development.js"></script>
<!--引入babel 用于Jsx转换称js-->
<script type="text/javascript" src="https://cdn.bootcss.com/babel-standalone/6.26.0/babel.min.js"></script>
<!--引入prop-->
<script type="text/javascript" src="https://cdn.bootcss.com/prop-types/15.6.1/prop-types.js"></script>
<script type="text/babel">
//1、创建组件
class Life extends React.Component{
state = {opacity:1};
/**1、组件挂载完毕时执行 */
componentDidMount(){
this.timer = setInterval(()=>{
console.log('@');
let {opacity} = this.state;
opacity -=0.1
if(opacity<=0) opacity=1
this.setState({opacity});
},200)
}
/**2、触发移除控件 */
delCompoent = ()=>{
ReactDOM.unmountComponentAtNode(document.getElementById('test'));
}
/**3、清除定时器 */
componentWillUnmount(){
clearInterval(this.timer);
}
render(){
return(
<div>
<h2 style={{opacity:this.state.opacity}}>我是一段渐变文字</h2>
<button onClick={this.delCompoent}>删除控件</button>
</div>
)
}
}
//2、渲染虚拟DOM到页面
ReactDOM.render(<Life />,document.getElementById('test'))
/*
1、React 解析组件<MyCommponment/>
2、发现组件是类式定义,随后进行实例化,调用里面上的render,返回虚拟的DOM
3、虚拟的DOM转为真实的DOM,随后呈现在页面中
*/
</script>
</body>
</html>
二、16以下版本的生命周期
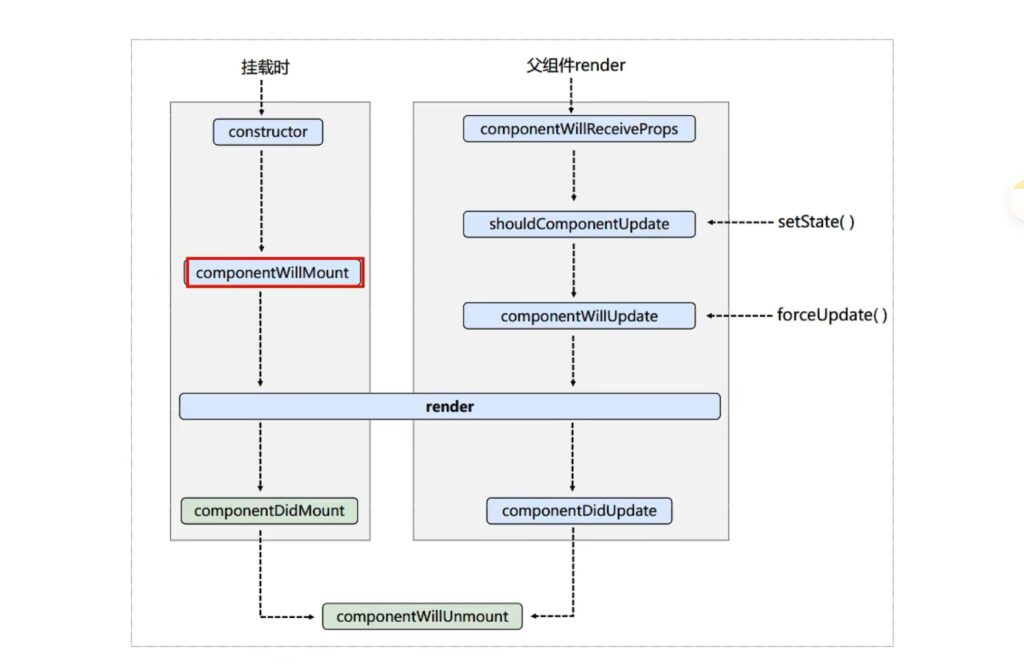
2.1挂载时的生命周期
- constructor:构造器
- componentWillMount:组件将要挂载时
- render:
- componentDidMount:组件完成挂载时
- componentWillUnmount:组件准备卸载时
<!Doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello World</title>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="test"></div>
<!--引入React 核心库-->
<script type="text/javascript" src="https://cdn.bootcss.com/react/16.4.0/umd/react.development.js"></script>
<!--引入React-dom 用于支持React操作DOM-->
<script type="text/javascript" src="https://cdn.bootcss.com/react-dom/16.4.0/umd/react-dom.development.js"></script>
<!--引入babel 用于Jsx转换称js-->
<script type="text/javascript" src="https://cdn.bootcss.com/babel-standalone/6.26.0/babel.min.js"></script>
<!--引入prop-->
<script type="text/javascript" src="https://cdn.bootcss.com/prop-types/15.6.1/prop-types.js"></script>
<script type="text/babel">
//1、创建组件
class Life extends React.Component{
//构造器
constructor(props){
console.log("1、count-constructor");
super(props);
this.state = {count:0};
}
//组件将要挂载时
componentWillMount(){
console.log("2、count-componentWillMount");
}
//组件完成挂载时
componentDidMount(){
console.log("4、count-componentDidMount");
}
addCount = ()=>{
const {count} = this.state;
this.setState({count:count+1})
}
delCompoent = ()=>{
console.log("Start-delCompoent");
ReactDOM.unmountComponentAtNode(document.getElementById('test'));
}
componentWillUnmount(){
console.log("count-componentWillUnmount");
}
render(){
const {count} = this.state;
console.log("3、render");
return(
<div>
<h2>当前求和为:{count}</h2>
<button onClick={this.addCount}>点我+1</button>
<button onClick={this.delCompoent}>卸载组件</button>
</div>
)
}
}
//2、渲染虚拟DOM到页面
ReactDOM.render(<Life />,document.getElementById('test'))
/*
1、React 解析组件<MyCommponment/>
2、发现组件是类式定义,随后进行实例化,调用里面上的render,返回虚拟的DOM
3、虚拟的DOM转为真实的DOM,随后呈现在页面中
*/
</script>
</body>
</html>
2.2完整的挂载生命周期
<!Doctype html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Hello World</title>
</head>
<body>
<!-- 准备好一个容器 -->
<div id="test"></div>
<!--引入React 核心库-->
<script type="text/javascript" src="https://cdn.bootcss.com/react/16.4.0/umd/react.development.js"></script>
<!--引入React-dom 用于支持React操作DOM-->
<script type="text/javascript" src="https://cdn.bootcss.com/react-dom/16.4.0/umd/react-dom.development.js"></script>
<!--引入babel 用于Jsx转换称js-->
<script type="text/javascript" src="https://cdn.bootcss.com/babel-standalone/6.26.0/babel.min.js"></script>
<!--引入prop-->
<script type="text/javascript" src="https://cdn.bootcss.com/prop-types/15.6.1/prop-types.js"></script>
<script type="text/babel">
//1、创建组件
//#region
class count extends React.Component{
//构造器
constructor(props){
console.log("1、count-constructor");
super(props);
this.state = {count:0};
}
//组件将要挂载时
componentWillMount(){
console.log("2、count-componentWillMount");
}
//组件完成挂载时
componentDidMount(){
console.log("4、count-componentDidMount");
}
addCount = ()=>{
const {count} = this.state;
this.setState({count:count+1})
}
delCompoent = ()=>{
console.log("Start-delCompoent");
ReactDOM.unmountComponentAtNode(document.getElementById('test'));
}
componentWillUnmount(){
console.log("count-componentWillUnmount");
}
/** 父组件更新部分*/
render(){
const {count} = this.state;
console.log("3、render");
return(
<div>
<h2>当前求和为:{count}</h2>
<button onClick={this.addCount}>点我+1</button>
<button onClick={this.delCompoent}>卸载组件</button>
</div>
)
}
}
class A extends React.Component{
constructor(props){
console.log("1、A-constructor");
super(props);
this.state = {carName:"宝马"};
}
//组件将要挂载时
componentWillMount(){
console.log("2、A-componentWillMount");
}
//组件完成挂载时
componentDidMount(){
console.log("4、A-componentDidMount");
}
shouldComponentUpdate(){
console.log("1、A-Click-shouldComponentUpdate");
return true;
}
componentWillUpdate(){
console.log("2、A-click-componentWillUpdate");
return true;
}
componentDidUpdate(){
console.log("4、A-click-componentDidUpdate");
return true;
}
changeCar =()=>{
this.setState({carName:"奥迪"})
}
render(){
console.log("3、A-render")
return (
<div>
<div>我是A组件,当前的车是{this.state.carName}</div>
<button onClick={this.changeCar}>换车</button>
<B carName={this.state.carName} />
</div>
)
}
}
class B extends React.Component{
constructor(props){
console.log("1、A-constructor");
super(props);
this.state = {carName:"宝马"};
}
//组件将要挂载时
componentWillMount(){
console.log("2、B-componentWillMount");
}
//组件完成挂载时
componentDidMount(){
console.log("4、B-componentDidMount");
}
/*状态变更时*/
componentWillReceiveProps(props){
console.log(props)
console.log("1、A-change-B-componentWillReceiveProps");
}
shouldComponentUpdate(){
console.log("2、A-change-B-shouldComponentUpdate");
return true;
}
componentWillUpdate(){
console.log("3、A-change-B-componentWillUpdate");
return true;
}
componentDidUpdate(){
console.log("5、A-change-B-componentDidUpdate");
return true;
}
render(){
console.log("3、B-render")
return (
<div>我是B组件,接收到的车是{this.props.carName}</div>
)
}
}
//2、渲染虚拟DOM到页面
ReactDOM.render(<A />,document.getElementById('test'))
/*
1、React 解析组件<MyCommponment/>
2、发现组件是类式定义,随后进行实例化,调用里面上的render,返回虚拟的DOM
3、虚拟的DOM转为真实的DOM,随后呈现在页面中
*/
</script>
</body>
</html>
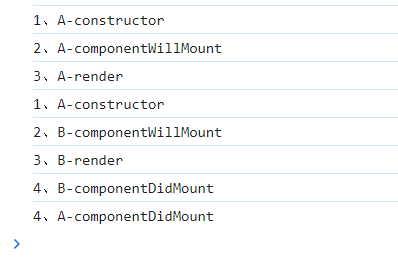
挂载时的顺序
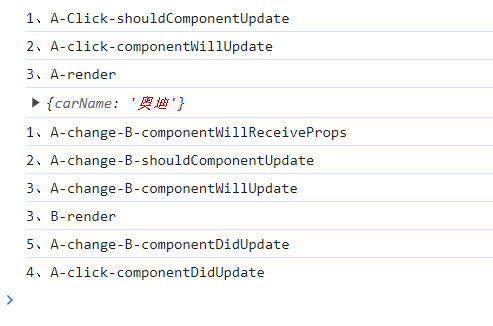
更新状态后的顺序
三、16以上版本的生命周期
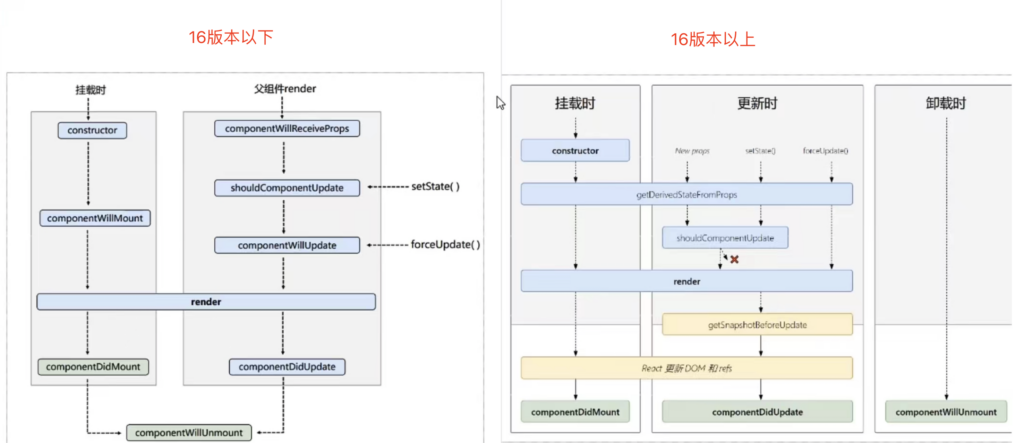